The PHP MVC (Model-View-Controller) design pattern is a architectural pattern that separates the application logic into three interconnected components – Model, View, and Controller. 1. Model: The model represents the data and business logic of the application. It handles the data storage, retrieval, and manipulation. In PHP, the model is typically implemented as classes that …
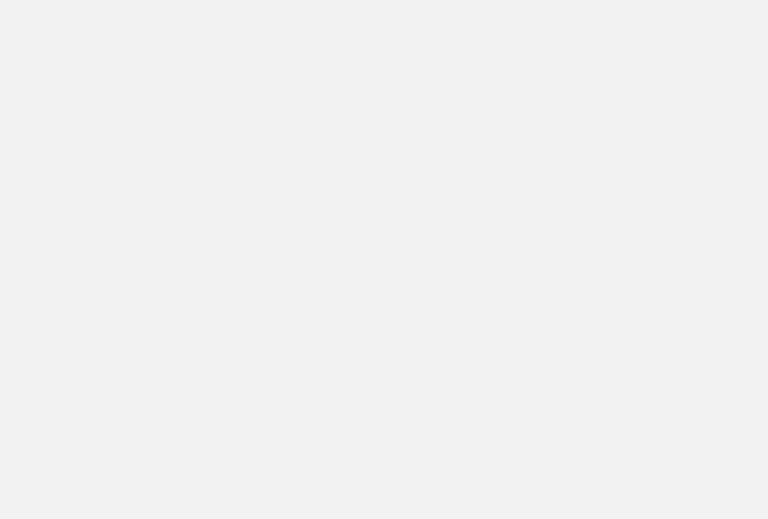