Session management in PHP is the process of maintaining the state and data of a user session across multiple requests. Here are some key points to consider when working with PHP session management: 1. Starting a session: To start a session, you need to call the `session_start()` function at the beginning of your PHP script. …
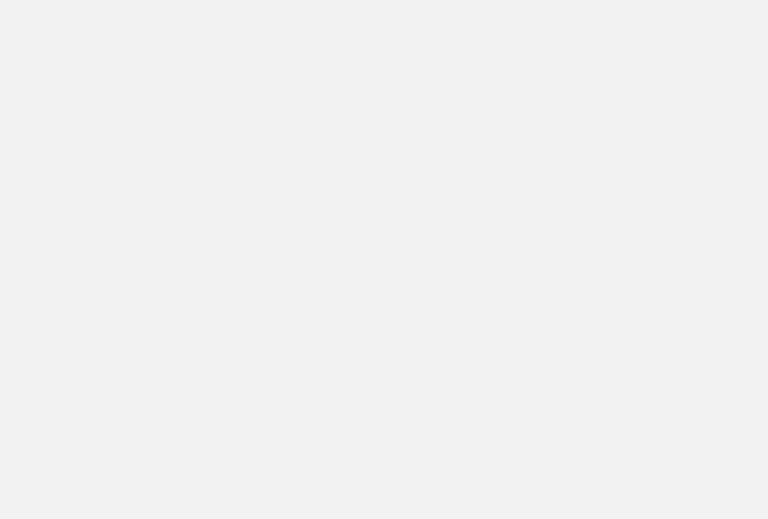