In PHP, an array is a variable that can hold multiple values of any data type. To create an array, you can use the array() function or the shorthand syntax []. Here are examples of creating arrays: “`php // Using array() function $fruits = array(“apple”, “banana”, “orange”); // Using shorthand syntax $fruits = [“apple”, “banana”, …
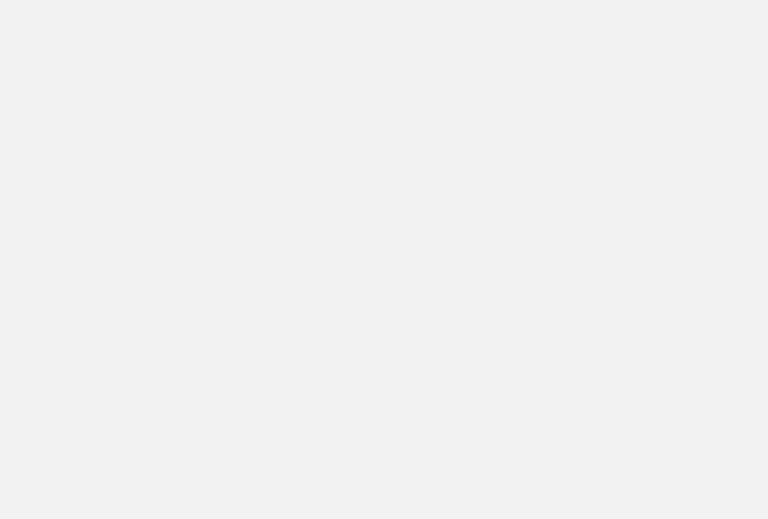