Here are some performance optimization techniques that can be applied to C# code: 1. Use efficient data structures: Choose the right data structure for the problem at hand. For example, use a HashSet instead of a List for fast membership checks, or use a Dictionary instead of a List when looking up values by key. …
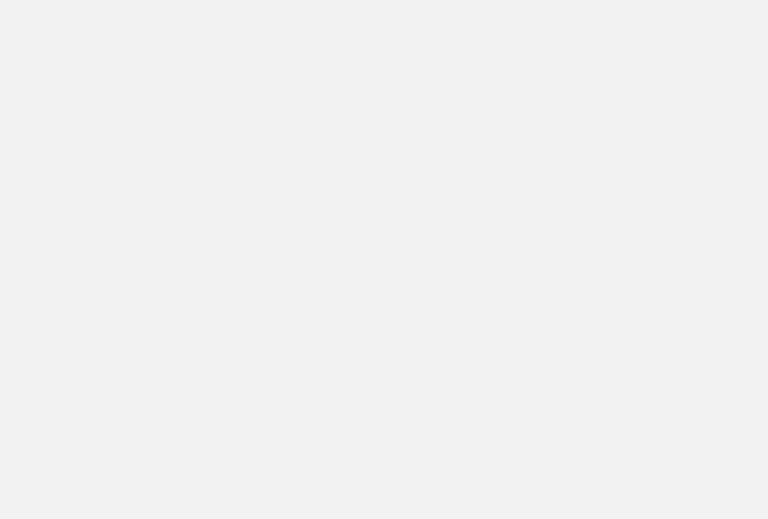