1. Opening a file: To open a file in PHP, you can use the `fopen()` function. This function takes two parameters – the name of the file and the mode in which to open the file (e.g., read, write, append). Example: “`php $file = fopen(“example.txt”, “r”); “` 2. Reading a file: To read the contents …
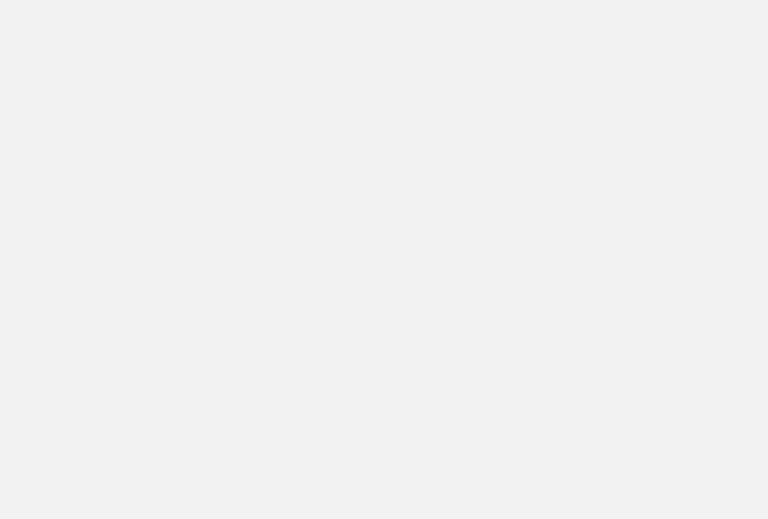