Cron is a time-based job scheduler in Unix-like operating systems like Linux. It allows you to schedule tasks (cron jobs) to run at specific intervals or times. You can use cron jobs to automate tasks like updating a database, generating reports, or sending scheduled emails. To set up a cron job with PHP, you can …
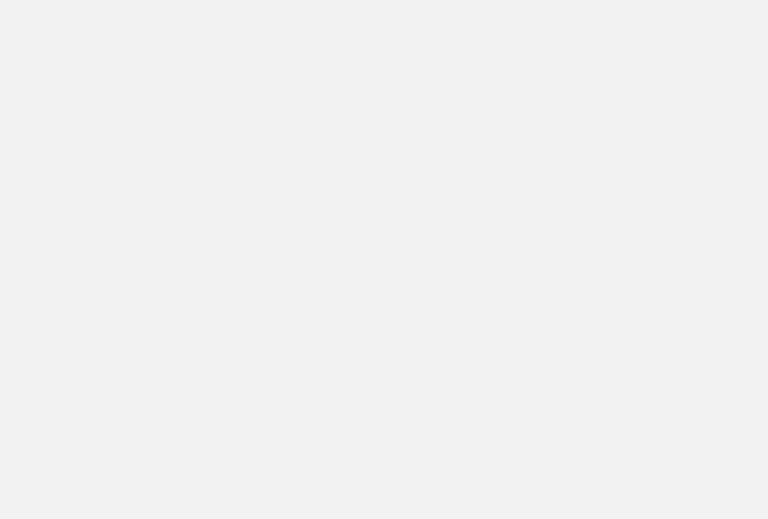