In PHP, you can use the built-in SOAP extension to consume SOAP web services. To call a SOAP web service in PHP, you’ll need to do the following: 1. Enable the SOAP extension in PHP by uncommenting the line `extension=soap` in your php.ini file. 2. Create a new instance of the `SoapClient` class, passing the …
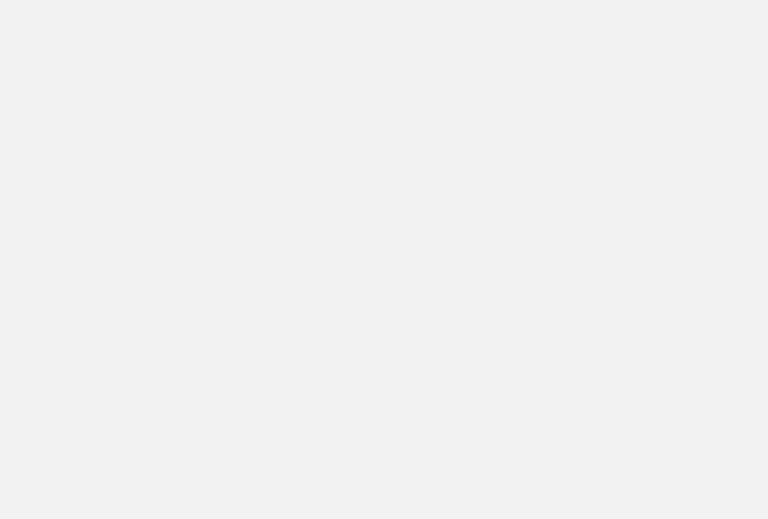