Arrays and collections are data structures that can store multiple values in a single variable. They are useful when you need to work with multiple values of the same type. Arrays: – Arrays are fixed in size and have a specific order. – You can access individual elements with an index. – Arrays can be …
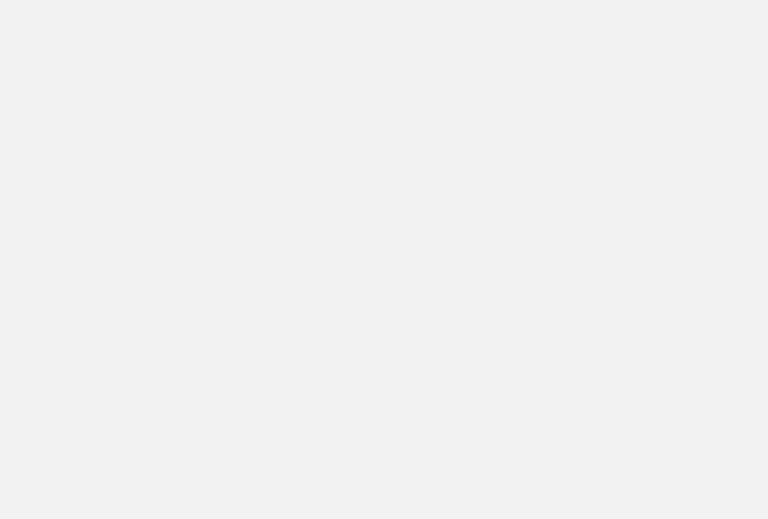